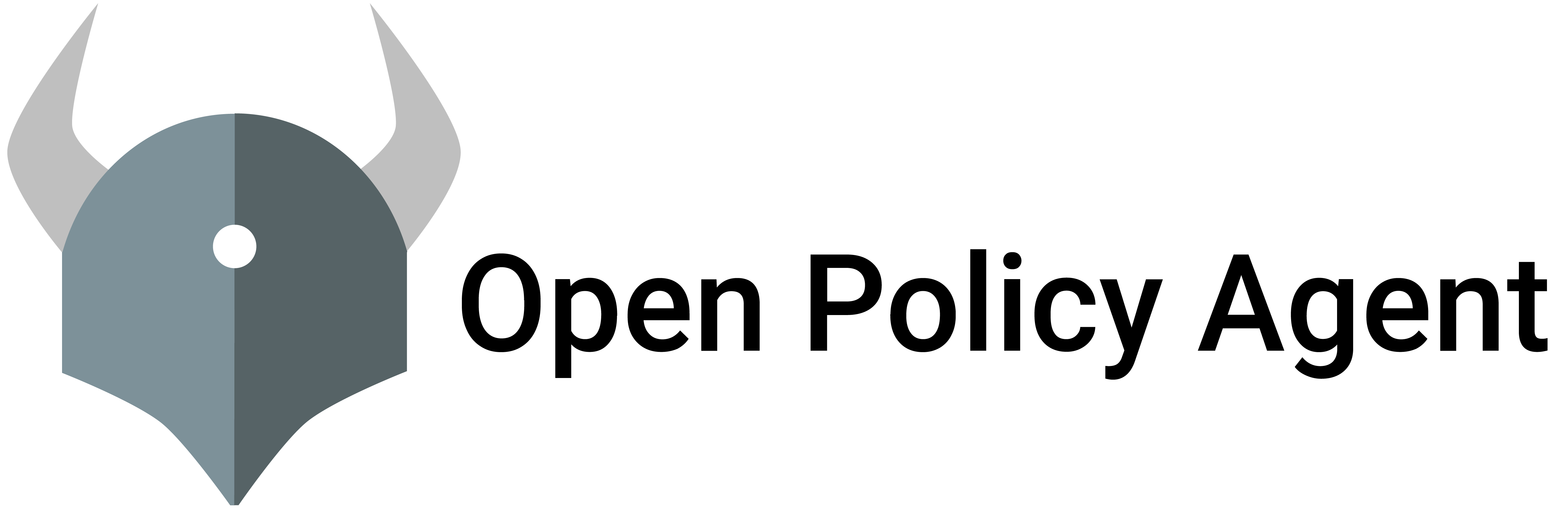
OPA is a policy engine that streamlines policy management across your stack for improved development, security and audit capability.
{}
{
"account": {
"state": "open"
},
"user": {
"risk_score": "low"
},
"transaction": {
"amount": 950
}
}
# Run your first Rego policy!
package payments
default allow := false
allow if {
input.account.state == "open"
input.user.risk_score in ["low", "medium"]
input.transaction.amount <= 1000
}
# Open in the Rego Playground to see the full example.
Created by
OPA is now maintained by Styra and a large community of contributors.
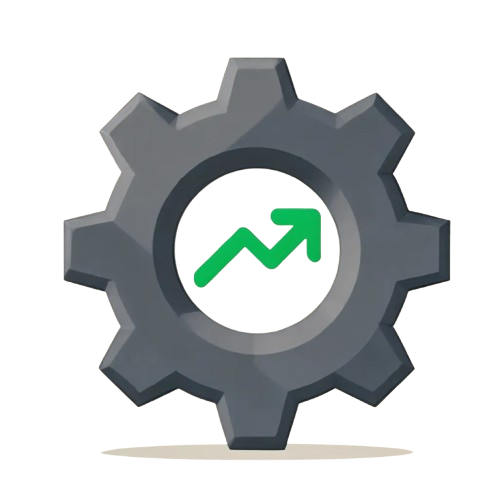
Developer Productivity: OPA helps teams focus on delivering business value by decoupling policy from application logic. Security & platform teams centrally manage shared policies, while developer teams extend them as needed within the policy system.
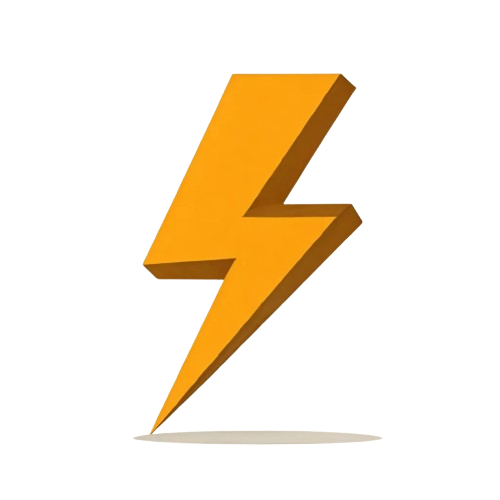
Performance: Rego, our domain-specific policy language, is built for speed. By operating on pre-loaded, in-memory data, OPA acts as a fast policy decision point for your applications.
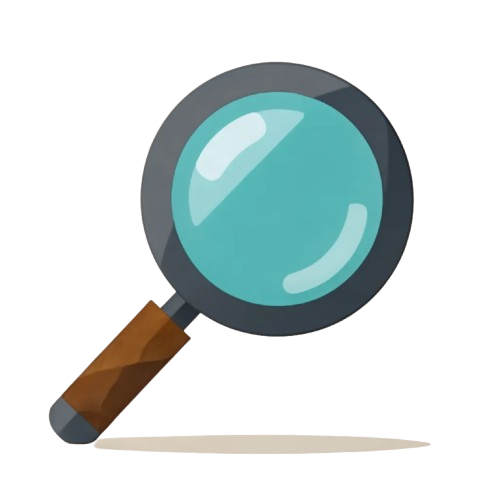
Audit & Compliance: OPA generates comprehensive audit trails for every policy decision. This detailed history supports auditing and compliance efforts and enables decisions to be replayed for analysis or debugging.
Interested to see more? Checkout the Maintainer Track Session from KubeCon.
Context-aware, Expressive, Fast, Portable
OPA is a general-purpose policy engine that unifies policy enforcement across the stack. OPA provides a high-level declarative language that lets you specify policy for a wide range of use cases. You can use OPA to enforce policies in applications, proxies, Kubernetes, CI/CD pipelines, API gateways, and more.
- API
- Envoy
- Kubernetes
- GenAI
Applications can directly integrate with OPA using our SDKs or REST API. This is great when your application needs to make domain specific runtime decisions.
package application.authz
# Only owner can update the pet's information. Ownership
# information is provided as part of the request data from
# the application.
default allow := false
allow if {
input.method == "PUT"
some petid
input.path = ["pets", petid]
input.user == input.owner
}
{
"role": "staff",
"owner": "bob@example.com",
"path": [
"pets",
"pet113-987"
],
"user": "alice@example.com"
}
{}
OPA has a native integration with the Envoy External Authorization API. This example shows a simple policy using the input document supplied from that API to authorize requests.
Browse more Envoy Examples on the playground.
package envoy.http.public
headers := input.attributes.request.http.headers
default allow := false
allow if {
input.attributes.request.http.method == "GET"
input.attributes.request.http.path == "/"
}
allow if headers.authorization == "Basic charlie"
{
"attributes": {
"request": {
"http": {
"headers": {
"authorization": "Basic bob"
},
"method": "GET",
"path": "/",
"protocol": "HTTP/1.1"
}
}
}
}
{}
OPA can be used to control which Kubernetes resources can be created in a given cluster. By configuring the Kubernetes API to send admission requests to OPA, you can create custom policies to enforce your organization's rules.
Browse more Kubernetes Examples on the playground.
package kubernetes.validating.existence
deny contains msg if {
value := input.request.object.metadata.labels.costcenter
not startswith(value, "cccode-")
msg := sprintf("Costcenter must start `cccode-`; found `%v`", [value])
}
deny contains msg if {
not input.request.object.metadata.labels.costcenter
msg := "Every resource must have a costcenter label"
}
{
"kind": "AdmissionReview",
"request": {
"kind": {
"kind": "Pod",
"version": "v1"
},
"object": {
"metadata": {
"name": "myapp",
"labels": {
"costcenter": "engineering"
}
},
"spec": {
"containers": [
{
"image": "nginx",
"name": "nginx-frontend"
},
{
"image": "mysql",
"name": "mysql-backend"
}
]
}
}
}
}
{}
Controlling access to generative AI endpoints can be complicated. With OPA, you can create custom rules to grant or deny access to specific users in your organization.
Browse more AI API Examples on the playground.
package ai.chat
deny contains message if {
every pattern in all_accessible_models {
not regex.match(pattern, input.parsed_body.model)
}
message := sprintf(
"Model '%s' is not in your accessible models: %s",
[input.parsed_body.model, concat(", ", all_accessible_models)],
)
}
# model_access is a mapping of role to patterns which match models
# that users might be accessing.
model_access := {
"interns": {"model-1"},
"testers": {"model-1", `^model-\d+-stage$`},
"data-analysts": {"model-1", `^model-\d+-internal$`},
}
all_accessible_models contains m if {
some group in input.groups
some m in model_access[group]
}
{
"user": {
"email": "alice@example.com",
"groups": [
"testers"
]
},
"parsed_body": {
"model": "model-2",
"messages": [
{
"role": "user",
"content": "Tell me about OPA"
}
]
}
}
{}